Hey Julien,
I’m sorry for the late ressponse, sorry I didn’t want to say “by segmentation” the real segmentation that we use in the medical field, It’s just a reshaping of the VTK surface to be identic to the VTI volume at a certain depth, like the video there,
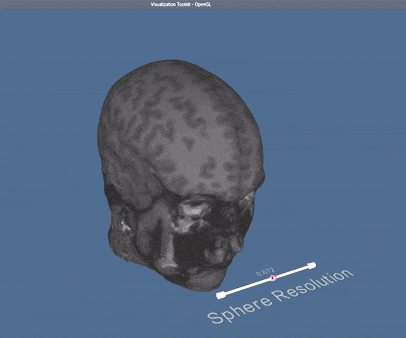
For the pipeline (C++) to do so:
- We charge a .nii MRI and a Skin VTK (a human head)
- We get the bounds (bounds[6]) from the nii reader.
- We calculate sizes:
xSize = bounds[1] - bounds[0];
ySize = bounds[3] - bounds[2];
zSize = bounds[5] - bounds[4];
- We create a vtkPlane that we set its Origin values to Sizes
vtkSmartPointer<vtkPlane> plane = vtkSmartPointer<vtkPlane>::New();
plane->SetOrigin(xSize/2.0,ySize/2.0,zSize/2.0);
plane->SetNormal(0.0, 0.0, 1.0);
- We create a vtkDecimatePro that we set Input Connection to the Output of skin VTK reader
vtkSmartPointer<vtkDecimatePro> deci=vtkSmartPointer<vtkDecimatePro>::New();
deci->SetInputConnection(vtkReader->GetOutputPort());
deci->SetTargetReduction(0.6);
deci->PreserveTopologyOn();
- We create a vtkSmoothPolyDataFilter that we set its Input Connection to vtkDecimatePro Outport
vtkSmartPointer
smoother=vtkSmartPointer<vtkSmoothPolyDataFilter>::New();
smoother->SetInputConnection(deci->GetOutputPort());
smoother->SetRelaxationFactor(0.8);
smoother->SetNumberOfIterations(100);
smoother->BoundarySmoothingOff();
smoother->FeatureEdgeSmoothingOff();
- We create a vtkTransform that we set its Translate to Sizes/2
vtkSmartPointer<vtkTransform> transform = vtkSmartPointer<vtkTransform>::New();
transform->Identity();
transform->PostMultiply();
transform->Translate(xSize/2.0,ySize/2.0,zSize/2.0);
transform->Scale(1,1,1);
transform->Translate(xSize/2.0,ySize/2.0,zSize/2.0);
- We create vtkTransformPolyDataFilter that we set its Input Connection to vtkSmoothPolyDataFilter and its Transform to vtkTransform:
vtkSmartPointer<vtkTransformPolyDataFilter> transformFilter = vtkSmartPointer<vtkTransformPolyDataFilter>::New();
transformFilter->SetInputConnection(smoother->GetOutputPort());
transformFilter->SetTransform(transform);
transformFilter->Update();
- Here where we use vtkProbeFilter :
vtkSmartPointer<vtkProbeFilter> probe = vtkSmartPointer<vtkProbeFilter>::New();
probe->SetInputConnection(transformFilter->GetOutputPort());
probe->SetSourceData(niiReader->GetOutput());
probe->SpatialMatchOn();
probe->Update();
vtkSmartPointer<vtkPolyDataMapper> map = vtkSmartPointer<vtkPolyDataMapper>::New();
map->SetInputConnection(probe->GetOutputPort());
map->SetScalarRange(probe->GetOutput()->GetScalarRange());
vtkSmartPointer<vtkActor> actor = vtkSmartPointer<vtkActor>::New();
actor->SetMapper(map);