Great appreciate the examples. I think the code is working so far but some small problems still exist such as
- Point distance is large in some region (we can still see some wide space between points in the edge). For some dense point cloud, this problem is more obvious and I am thinking another method to first reconstruct the point cloud surface and assign the color. But it seems not working well, as shown in fig. 1.
- I try with generating with the mesh with point index and triangular mesh, but it seems not working well and I am not sure if this is the problem that I didn’t change the property of the mesh, as shown in fig. 2.
- When we get close to the object, we can see the artifacts on the dense mesh grid. I am thinking maybe using OpenGL texture mapping might be another possible solution?
- I am thinking about using stl and look up table to assign the color vector to the points.
Any improvements are welcomed. Thanks again.
I have finished the code:
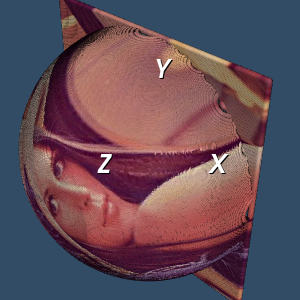
# Load the data object
‘’’
pcd: object of pcd
pc: point coordinates, M x 3
pc_color: color vectors, M x 3
img_color: color image for textured mapping
‘’’
test = BTL_GL.BTL_GL()
pcd, pc, pc_color, MeshGrid, img_color = test.GL_NonFlat()
# Define the points polydata
points = vtk.vtkPoints()
for i in range(len(pc)):
points.InsertNextPoint(pc[i, 0], pc[i, 1], pc[i, 2])
polydata = vtk.vtkPolyData()
polydata.SetPoints(points)
# Define the color to the polydata
colors = vtk.vtkUnsignedCharArray()
colors.SetNumberOfComponents(3)
colors.SetNumberOfTuples(polydata.GetNumberOfPoints())
for i in range(len(pc_color)):
colors.InsertTuple3(i, pc_color[i, 0], pc_color[i, 1], pc_color[i, 2])
# Connect the point object to the color object
polydata.GetPointData().SetScalars(colors)
# Define the VertexGlyphFilter
vgf = vtk.vtkVertexGlyphFilter()
vgf.SetInputData(polydata)
vgf.Update()
pcd = vgf.GetOutput()
# Define the mapper
point_mapper = vtk.vtkPolyDataMapper()
point_mapper.SetInputData(pcd)
# Define the actor
actor = vtk.vtkActor()
actor.SetMapper(point_mapper)
actor.GetProperty().SetPointSize(10)
actor.GetProperty().RenderPointsAsSpheresOn()
ren = vtk.vtkRenderer()
ren.SetBackground(.2, .3, .4)
ren.AddActor(actor)
renWin = vtk.vtkRenderWindow()
renWin.AddRenderer(ren)
# Interactor
renderWindowInteractor = vtk.vtkRenderWindowInteractor()
renderWindowInteractor.SetRenderWindow(renWin)
# Begin Interaction
renWin.Render()
renderWindowInteractor.Start()