There are several tests and examples for each VTK filter. You can also search on google or github and expect to find thousands of usage examples in various projects. If any details of the inner working of the filter are not clear then you can have a look at the source code. If you want to have a general overview of VTK and most filters, you can read the VTK textbook.
If you don’t want to spend time with googling then you can get a fairly comprehensive answer from chatgpt about most VTK related questions. For example, you can get all the necessary code by asking these questions:
- How to merge multiple vtkPolyData and display each with a single actor in different colors?
- How to assign the same cell data to all cells in a vtkPolyData?
- Provide sample code that sets scalar value not just for the first cell but for all the cells.
This is the fully functional code that you can put together by merging the answers that ChatGPT provided
import vtk
# Create some example polydata
polydata1 = vtk.vtkPolyData()
points1 = vtk.vtkPoints()
points1.InsertNextPoint(0, 0, 0)
points1.InsertNextPoint(1, 0, 0)
points1.InsertNextPoint(0, 1, 0)
points1.InsertNextPoint(1, 1, 0)
polydata1.SetPoints(points1)
triangle1 = vtk.vtkTriangle()
triangle1.GetPointIds().SetId(0, 0)
triangle1.GetPointIds().SetId(1, 1)
triangle1.GetPointIds().SetId(2, 2)
cells1 = vtk.vtkCellArray()
cells1.InsertNextCell(triangle1)
polydata1.SetPolys(cells1)
# Create a vtkIntArray with a single value
cell_data1 = vtk.vtkIntArray()
cell_data1.SetName("ColorIndex")
cell_data1.SetNumberOfValues(polydata1.GetNumberOfCells())
cell_data1.FillComponent(0, 0)
polydata1.GetCellData().SetScalars(cell_data1)
polydata2 = vtk.vtkPolyData()
points2 = vtk.vtkPoints()
points2.InsertNextPoint(1, 0, 0)
points2.InsertNextPoint(2, 0, 0)
points2.InsertNextPoint(1, 1, 0)
points2.InsertNextPoint(2, 1, 0)
polydata2.SetPoints(points2)
triangle2 = vtk.vtkTriangle()
triangle2.GetPointIds().SetId(0, 0)
triangle2.GetPointIds().SetId(1, 1)
triangle2.GetPointIds().SetId(2, 2)
cells2 = vtk.vtkCellArray()
cells2.InsertNextCell(triangle2)
polydata2.SetPolys(cells2)
# Create a vtkIntArray with a single value
cell_data2 = vtk.vtkIntArray()
cell_data2.SetName("ColorIndex")
cell_data2.SetNumberOfValues(polydata1.GetNumberOfCells())
cell_data2.FillComponent(0, 1)
polydata2.GetCellData().SetScalars(cell_data2)
# Combine the polydata using vtkAppendPolyData
append = vtk.vtkAppendPolyData()
append.AddInputData(polydata1)
append.AddInputData(polydata2)
append.Update()
# Create a lookup table and set colors for each component
lut = vtk.vtkLookupTable()
lut.SetNumberOfTableValues(2)
lut.SetTableValue(0, 1.0, 0.0, 0.0, 1.0) # Red for first component
lut.SetTableValue(1, 0.0, 1.0, 0.0, 1.0) # Green for second component
# Create a mapper and actor for the combined polydata
mapper = vtk.vtkPolyDataMapper()
mapper.SetLookupTable(lut)
mapper.SetInputData(append.GetOutput())
mapper.SetScalarRange(0, 1) # Set the range of the lookup table
actor = vtk.vtkActor()
actor.SetMapper(mapper)
# Create a renderer, window, and interactor to display the actor
renderer = vtk.vtkRenderer()
render_window = vtk.vtkRenderWindow()
render_window.AddRenderer(renderer)
interactor = vtk.vtkRenderWindowInteractor()
interactor.SetRenderWindow(render_window)
renderer.AddActor(actor)
interactor.Initialize()
render_window.Render()
interactor.Start()
Execution result (multiple polydata merged and displayed in different colors):
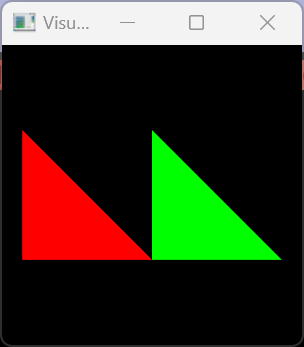
ChatGPT is not perfect in that it often does not provide the exact code you need on the first question but it is very close and with a few clarifications you can get all the code you need. I would say it is now at the level of a quite experienced VTK user that is just a bit too busy to read your questions very thoroughly. Google should be really panicking now.